JavaScript Simple Projects With Source Code - Temperature Converter
Last Updated: 2023-03-21 01:23:33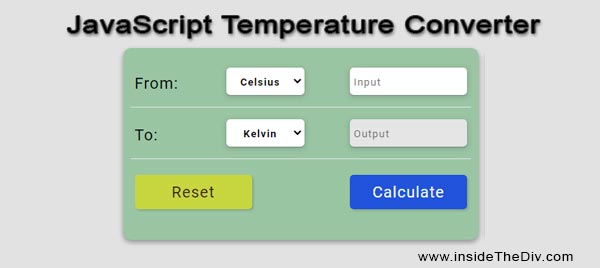
In this blog post, we will discuss how to build a JavaScript Simple Projects Temperature converter project with source code. This project is perfect for beginners who are learning Javascript and want to apply their skills to a real-world project.
What is a JavaScript Simple Projects Temperature Converter?
A temperature converter is a tool that is used to convert temperature from one unit of measurement to another. For example, it can convert a temperature in degrees Celsius to degrees Fahrenheit, or vice versa. Some temperature calculators may also allow you to convert temperatures to Kelvin. The conversion between these units is determined by a set of mathematical formulas. The use of a temperature calculator can be useful for various applications, including scientific experiments, cooking, and weather monitoring.
A JavaScript temperature converter is a program or function that allows users to convert temperatures between different units of measurement using JavaScript programming language. It can be created as a web application or a stand-alone program.
You can handle conversion between the three most commonly used temperature units: Celsius, Fahrenheit, and Kelvin. It can also handle conversion to and from other temperature units, such as Rankine and Réaumur.
If you are a beginner and want to learn javascript from basic, please check out our other JavaScript Simple Projects With Source Code
- Javascript Tip calculator
- Javascript Length Converter
- Javascript Percentage Calculator
- Javascript Fraction Calculator
- Javascript BMI Calculator
- Javascript Speed Calculator
Use of JavaScript Simple Projects Temperature Converter
A temperature converter is a useful tool that can be used in many different settings. Here are a few examples of how a temperature converter can be used:
- Cooking: When following a recipe that specifies a temperature in Celsius or Fahrenheit, it may be helpful to convert the temperature to the other unit of measurement in order to use an oven or stovetop that is calibrated in a different unit.
- Weather forecasting: Temperature is an important variable in weather forecasting. A temperature converter can be used to convert temperatures between Celsius and Fahrenheit so that weather reports can be understood by people who are accustomed to different units of measurement.
- Science and engineering: Temperature is an important variable in many scientific and engineering applications. A temperature converter can be used to convert temperatures between different units of measurement, such as Celsius and Kelvin, in order to perform calculations and make accurate measurements.
- Travel: When traveling to a country that uses a different unit of temperature measurement, it can be useful to have a temperature converter to help understand weather reports and other temperature-related information.
- Education: A temperature converter can be a helpful tool in teaching students about different temperature units of measurement and how to convert between them. It can also be used in physics, chemistry, and other science classes to perform calculations that involve temperature.
Design JavaScript Simple Projects Temperature Converter in HTML CSS
To design a temperature converter in HTML and CSS, you will need to create a form that allows users to enter a temperature value and select the input and output units. Here are the basic steps to follow:
- Create an HTML form with the following elements:
A text input field for the temperature value
Two radio buttons or a drop-down menu to select the input unit (e.g. Celsius, Fahrenheit, Kelvin)
Two radio buttons or a drop-down menu to select the output unit (e.g. Celsius, Fahrenheit, Kelvin)
A button to submit the form
Style the form using CSS to make it visually appealing and user-friendly. You can use colors, fonts, and layouts to make the form easy to read and navigate. - Add JavaScript code to the form to perform the temperature conversion when the user submits the form. You can write a function that takes in the input temperature and unit, the output unit, and applies the appropriate conversion formula to convert the temperature.
- Display the converted temperature to the user in a visually pleasing way, such as in a div element on the page.
- Test the form to ensure that it is working properly and accurately converting temperatures between units of measurement.
Overall, the design should be clean, simple, and easy to use. Users should be able to enter a temperature value and select the input and output units in a straightforward way, and the results of the conversion should be clear and easy to understand.
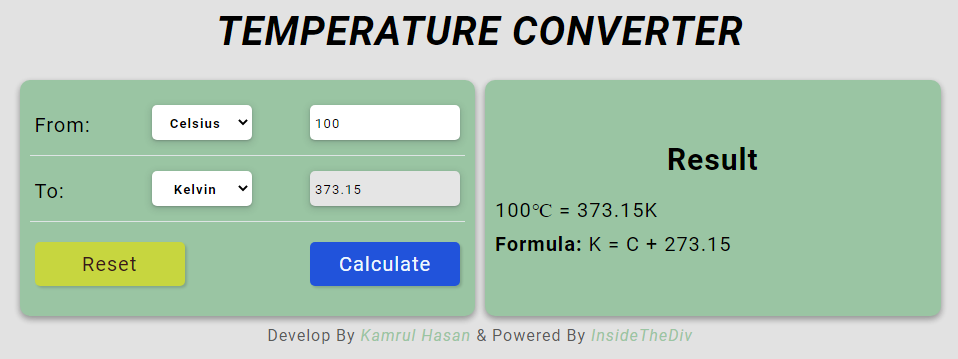
< div id="calculator">
< form>
< input type="text" id="temperature" placeholder="Enter temperature">
< select id="unit">
< option value="celsius">Celsius< /option>
< option value="fahrenheit">Fahrenheit< /option>
< option value="kelvin">Kelvin< /option>
< /select>
< button id="convert">Convert< /button>
< button id="reset">Reset< /button>
< /form>
< div id="result">< /div>
< /div>
The HTML structure consists of a div with an id of "calculator", which serves as the container for the calculator. Inside the div, there is a form with three elements: an input field for entering the temperature, a select menu for choosing the unit of measurement, and two buttons for converting the temperature and resetting the form.
JavaScript Simple Projects Temperature Converter Example Code
To create a JavaScript temperature converter, you can follow these basic steps:
- Define the function: Start by defining a function in JavaScript that will take in the input temperature, the input unit, and the desired output unit. You can name this function something like "temperatureConverter".
- Set up the conversion formulas: Inside the function, set up conversion formulas for each possible input and output unit. For example, to convert Celsius to Fahrenheit, the formula is (temperature * 1.8) + 32.
- Use conditional statements: Use conditional statements (if/else or switch statements) to determine which formula to use based on the input and output units selected by the user.
- Output the result: Once the correct conversion formula has been applied, output the result in a visually pleasing way, such as in a div element on the page.
- Test the function: Test the function to make sure it is working properly and accurately converting temperatures between different units of measurement.
Some additional tips to keep in mind when creating a JavaScript temperature converter:
- Make sure to validate user input to ensure that the temperature value is a number and that the selected units are valid.
- Use appropriate variable names to make the code easy to read and understand.
- Consider using a form to allow users to enter the temperature value and select the input and output units, or provide clear instructions on how to use the function in your application or website.
// convert temperature from Celsius to Fahrenheit
function celsiusToFahrenheit(celsius) {
return (celsius * 9 / 5) + 32;
}
// convert temperature from Fahrenheit to Celsius
function fahrenheitToCelsius(fahrenheit) {
return (fahrenheit - 32) * 5 / 9;
}
// get form elements
const temperatureInput = document.querySelector("#temperature");
const unitSelect = document.querySelector("#unit");
const convertButton = document.querySelector("#convert");
This JavaScript code first defines two functions, celsiusToFahrenheit and fahrenheitToCelsius, that perform the temperature conversion.
- The celsiusToFahrenheit function takes a temperature in Celsius as an argument and returns the equivalent temperature in Fahrenheit using the formula (celsius * 9 / 5) + 32.
- The fahrenheitToCelsius function takes a temperature in Fahrenheit as an argument and returns the equivalent temperature in Celsius using the formula (fahrenheit - 32) * 5 / 9.
- The code then gets the form elements using querySelector, including the temperature input, unit select, convert button, and result container.
- After that, we have to click the convert button and this button will call the convert method and show the result.
Temperature conversion formula list
The temperature conversion formula is a mathematical formula that is used to convert a temperature value from one unit of measurement to another. There are several common temperature scales used around the world, including Celsius (°C), Fahrenheit (°F), Kelvin (K), and Rankine (°R). The conversion formula for each of these scales depends on the starting and ending scales being used.
For example, to convert a temperature from Celsius to Fahrenheit, you can use the following formula:
Fahrenheit = (Celsius * 1.8) + 32
This formula takes the input temperature in Celsius and multiplies it by 1.8, then adds 32 to get the equivalent temperature in Fahrenheit. To convert a temperature from Fahrenheit to Celsius, the formula is:
Celsius = (Fahrenheit - 32) / 1.8
This formula subtracts 32 from the input temperature in Fahrenheit, then divides the result by 1.8 to get the equivalent temperature in Celsius.
Similarly, to convert a temperature from Celsius to Kelvin, the formula is:
Kelvin = Celsius + 273.15
To convert a temperature from Kelvin to Celsius, the formula is:
Celsius = Kelvin - 273.15
Other temperature conversion formulas exist for converting between Fahrenheit, Kelvin, and Rankine as well. It's important to remember that when performing temperature conversions, it's necessary to use the correct formula for the starting and ending scales being used to get an accurate result.
Convert from/to | Celsius (°C) | Fahrenheit (°F) | Kelvin (K) | Rankine (°R) |
---|---|---|---|---|
Celsius (°C) | N/A | (Celsius * 1.8) + 32 | Celsius + 273.15 | (Celsius + 273.15) * 1.8 |
Fahrenheit (°F) | (Fahrenheit - 32) / 1.8 | N/A | (Fahrenheit + 459.67) / 1.8 | Fahrenheit + 459.67 |
Kelvin (K) | Kelvin - 273.15 | Kelvin * 1.8 - 459.67 | N/A | Kelvin * 1.8 |
Rankine (°R) | (Rankine - 491.67) * 5/9 | Rankine - 459.67 | Rankine * 5/9 | N/A |
Conclusion
Creating a JavaScript Temperature Converter project is a great way for beginners to learn how to use JavaScript for simple mathematical calculations and user interactions. Through this project, beginners can learn about the basics of HTML, CSS, and JavaScript, as well as how to use event listeners, input fields, and output fields to create an interactive application that converts temperatures from one unit to another.
By building this project, beginners can gain valuable experience in developing simple applications and improve their understanding of programming concepts such as functions, variables, and basic logic. This project can also serve as a starting point for more complex web applications and can be expanded to include additional features such as different temperature scales, multiple input fields, and more advanced user interactions.
Still you face problems, feel free to contact with me, I will try my best to help you.