JavaScript Simple Projects With Source Code - BMI Calculator
Last Updated: 2023-03-12 18:44:51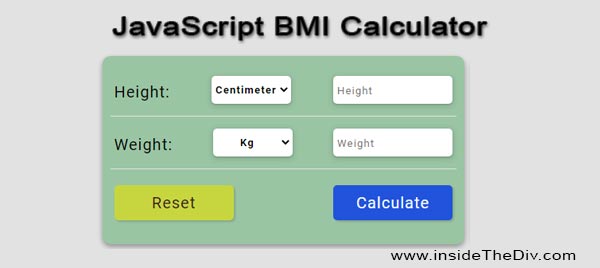
If you are a beginner looking to improve your javascript programming skills, developing JavaScript Simple Projects is one of the best ways. Now we will create a BMI (Body Mass Index) javascript project.
In this project, you will use HTML, CSS, and JavaScript to create a basic web page that allows users to input their height and weight and calculates their BMI. You will be applying simple mathematical formulas to calculate the BMI, and conditional statements to display the results in different categories based on the calculated value.
The source code for the BMI calculator project is readily available here and can be easily customized to suit your specific needs. By completing this project, you will have gained valuable experience in programming concepts such as input/output, arithmetic, conditional statements, and event handling.
JavaScript Simple Projects BMI Calculator
A BMI calculator is a tool used to estimate a person's body fat based on their height and weight. BMI (Body Mass Index) is a simple calculation that divides a person's weight in kilograms by their height in meters squared. The result is then used to classify the person into categories such as underweight, normal weight, overweight, or obese.
A JavaScript BMI to fraction calculator is a specific type of BMI calculator that not only calculates the BMI but also converts the decimal result to a fraction. This can be useful for people who prefer to express their BMI as a fraction instead of a decimal, especially in certain contexts such as the medical field.
By using JavaScript, a BMI to-fraction calculator can be created and embedded in a webpage, allowing users to easily input their height and weight and obtain a calculated result that is automatically converted to a fraction. This can be a useful tool for people who want to track their weight and body fat over time and monitor their overall health and fitness levels.
If you are a beginner and want to learn javascript from basic, please check out our other JavaScript Simple Projects With Source Code
- Javascript Tip calculator
- Javascript Temperature Converter
- Javascript Length Converter
- Javascript Percentage Calculator
- Javascript Fraction Calculator
- Javascript Speed Calculator
Use of JavaScript Simple Projects BMI Calculator
Javascript BMI calculator can be used by healthcare professionals to quickly evaluate a patient's weight status. It can also be used by individuals to monitor their own weight status.
The use of a BMI (Body Mass Index) calculator can be beneficial in several real-life scenarios. Here are some examples:
- Medical Consultations: Medical professionals use BMI as a screening tool to assess a patient's risk of certain health conditions related to excess body fat. A BMI calculator can be used to estimate an individual's BMI, which can help healthcare professionals provide targeted health advice.
- Fitness Tracking: People who are trying to maintain or lose weight can use a BMI calculator to track their progress. By inputting their height and weight regularly, they can monitor their BMI and make necessary adjustments to their fitness and diet plan.
- Sports and Athletics: Athletes often use BMI as a measure of body composition. For example, wrestlers may need to meet a certain BMI to qualify for a weight class.
- Weight Management Programs: Many weight management programs use BMI to determine eligibility and track progress. A BMI calculator can help individuals determine if they qualify for a particular program and track their progress throughout the program.
- Nutrition and Diet Planning: Dietitians and nutritionists use BMI as a tool for assessing their clients' nutritional status and developing a personalized plan to meet their health and fitness goals.
- Public Health Programs: Public health organizations may use BMI to assess the prevalence of obesity within a population or demographic group. This information can be used to design public health programs and policies aimed at improving overall health outcomes.
Design BMI calculator in HTML CSS
Designing a BMI (Body Mass Index) calculator for a web page can be done in a few simple steps using HTML, CSS, and JavaScript. Here is a step-by-step guide:
- Set up the HTML file: Create a new HTML file and add a form with two input fields, one for weight and the other for height. Add a submit button to trigger the BMI calculation.
- Create the CSS file: Add a CSS file to style the form and create a simple user interface. You can use basic CSS properties to define the look and feel of the form, such as colors, fonts, and layout.
- Add JavaScript for the BMI calculation: Use JavaScript to calculate the BMI based on the input values entered by the user. Write a function that takes the weight and height as parameters and returns the BMI value.
- Display the BMI result: Use JavaScript to display the calculated BMI value on the web page. You can add a new element to the HTML file to display the result and update it dynamically when the user submits the form.
- Add error handling: Validate the user's input and add error messages to alert the user if the input is invalid. For example, if the user enters a negative weight or height value, display an error message.
- Test and debug: Test the BMI calculator to make sure it is working correctly. Use the console to debug any errors or issues that may arise.
By following these steps, you can create a basic BMI calculator that can be easily customized and integrated into any web page.
< !DOCTYPE html>
< html>
< head>
< title>BMI Calculator< /title>
< link rel="stylesheet" href="style.css">
< /head>
< body>
< div id="bmi-form">
< h1>BMI Calculator< /h1>
< form>
< label>Height:< /label>
< input type="number" id="height" placeholder="Enter height">
< select id="height-unit">
< option value="inches">inches< /option>
< option value="centimeters">centimeters< /option>
< /select>
< br>
< label>Weight:< /label>
< input type="number" id="weight" placeholder="Enter weight">
< select id="weight-unit">
< option value="pounds">pounds< /option>
< option value="kilograms">kilograms< /option>
< /select>
< br>
< button type="button" id="calculate-btn">Calculate BMI< /button>
< /form>
< div id="bmi-result">< /div>
< /div>
< script src="script.js">< /script>
< /body>
< /html>
Here is the CSS design for the HTML page.
#bmi-form {
width: 400px;
margin: auto;
text-align: center;
background-color: #f5f5f5;
padding: 20px;
border-radius: 5px;
}
h1 {
font-size: 36px;
margin-top: 0;
margin-bottom: 30px;
}
label {
display: block;
margin-bottom: 10px;
text-align: left;
}
input[type="number"] {
width: 80px;
padding: 5px;
border-radius: 3px;
border: 1px solid #ccc;
margin-right: 10px;
}
select {
width: 100px;
padding: 5px;
border-radius: 3px;
border: 1px solid #ccc;
margin-left: 10px;
}
button {
margin-top: 20px;
background-color: #4CAF50;
color: white;
border: none;
border-radius: 5px;
padding: 10px 20px;
cursor: pointer;
}
#bmi-result {
margin-top: 20px;
font-size: 24px;
font-weight: bold;
}
JavaScript Simple Projects BMI Calculator Example Code
The JavaScript code for the BMI calculator takes the height and weight input from the user and converts the values to the metric system (if necessary),
To calculate BMI (Body Mass Index), you can use the formula:
BMI = weight(kg) / height(m)^2
Here are some examples of how to calculate BMI:
Example 1: A person who weighs 68 kg and is 1.75 meters tall.
BMI = 68 / (1.75^2) = 22.2
Example 2: A person who weighs 80 kg and is 1.80 meters tall.
BMI = 80 / (1.80^2) = 24.7
To write JavaScript code for a BMI calculator, here are some guidelines you can follow:
- Create a JavaScript function to calculate BMI.
- Add error handling to the JavaScript function to validate user input.
- Then write the JavaScript code for a BMI calculator is to create a function that will calculate the BMI. This function should take the weight and height values as inputs and return the calculated BMI.
- Next, you need to retrieve the weight and height values from the user input. You can do this by accessing the values of the input fields using their IDs.
- Next, you can call the calculate function with the input values to get the BMI. You can then display the result using an alert box or by adding an element to the HTML page.
- Finally, you can add an event listener to the form submit button to trigger the calculation.
- By following these steps, you can create a basic BMI calculator in JavaScript that can be easily integrated into a web page.
Calculates the BMI using the formula mentioned above. The code is as follows:
function calculateBMI() {
var height = parseFloat(document.getElementById("height").value);
var weight = parseFloat(document.getElementById("weight").value);
var heightUnit = document.getElementById("height-unit").value;
var weightUnit = document.getElementById("weight-unit").value;
if(heightUnit === "inches"){
height = height * 0.0254;
}
if(weightUnit === "pounds"){
weight = weight * 0.453592;
}
var BMI = weight / (height * height);
BMI = BMI.toFixed(2);
if(isNaN(BMI)){
document.getElementById("result").innerHTML = "Please enter valid height and weight values.";
}
else{
document.getElementById("result").innerHTML = "Your BMI is " + BMI;
}
}
How This Project Will Help Beginners To Learn Javascript
The BMI Calculator project can be a helpful learning tool for beginners who want to learn JavaScript. Here are a few ways in which it can help:
- Understanding basic syntax: Writing JavaScript code for a simple project like this can help beginners understand the basic syntax of the language. They can learn about variables, functions, and if/else statements, which are fundamental concepts in programming.
- Hands-on experience: Working on a project gives beginners hands-on experience with writing code. They can experiment with different parts of the code, see how the changes affect the output, and learn from their mistakes.
- Event handling: The BMI Calculator project involves event handling, which is an important concept in JavaScript. Beginners can learn about event listeners and how to handle events in the browser.
- Input validation: Validating user input is a key part of programming, and the BMI Calculator project provides an opportunity for beginners to practice this skill. They can learn how to check for errors and provide helpful feedback to the user.
- Real-world application: The BMI Calculator is a practical application of JavaScript that can be used in a real-world scenario. By working on this project, beginners can see how JavaScript can be used to solve everyday problems.
Overall, the BMI Calculator project can be an excellent way for beginners to learn JavaScript. By working on a simple project, they can gain a better understanding of the language and develop the skills they need to tackle more complex projects in the future.
Conclusion
In conclusion, the BMI Calculator is a simple yet practical JavaScript project that can help beginners learn the basics of the language. By creating a BMI calculator, beginners can gain hands-on experience with JavaScript syntax, event handling, input validation, and other important programming concepts. This project can be easily integrated into a web page, making it a great starting point for beginners who want to learn how to build functional applications with JavaScript. With a solid understanding of the fundamental concepts and hands-on experience with real-world scenarios, beginners can gain the confidence and skills they need to tackle more complex projects in the future.
Still you face problems, feel free to contact with me, I will try my best to help you.