JavaScript Simple Projects With Source Code - Percentage Calculator
Last Updated: 2023-03-09 00:10:26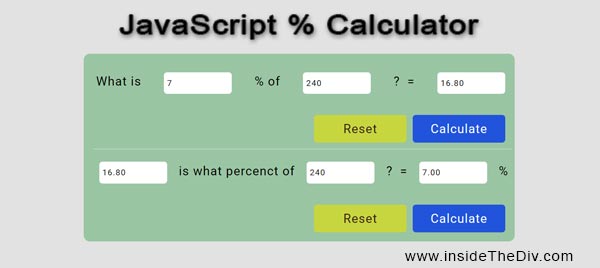
If you are a beginner and want to improve your javascript skill then building javascript Simple Projects is one of the best ways. You can create a simple percentage calculator in javascript.
A percentage calculator is a tool that is used to calculate the percentage value of a number or the percentage increase or decrease between two numbers. This tool can be used in various fields such as education, finance, and business to perform calculations easily and quickly.
JavaScript Percentage Calculator is a web-based tool that is built using JavaScript programming language. JavaScript is a popular client-side scripting language that is widely used for creating interactive and dynamic web pages. With JavaScript, developers can create powerful and responsive web applications that run on any browser.
In this article, we will provide the source code for a simple JavaScript Percentage Calculator project that is suitable for beginners. We will walk you through the steps to create the Percentage Calculator and explain the use of each code snippet.
If you are a beginner and want to learn javascript from basic, please check out our other JavaScript Simple Projects With Source Code
- Javascript Tip calculator
- Javascript Temperature Converter
- Javascript Length Converter
- Javascript Fraction Calculator
- Javascript BMI Calculator
- Javascript Speed Calculator
Use of javascript Simple Projects Percentage Calculator
Percentage Calculator can be used in various situations, some of them are as follows:
- In Education: In education, a Percentage Calculator is used to calculate the percentage marks obtained by a student in an exam.
- In Finance: In finance, a Percentage Calculator is used to calculate the percentage increase or decrease in the value of an investment.
- In Business: In business, a Percentage Calculator is used to calculate the percentage of profit or loss made by a company.
- In Sales and Marketing: In sales and marketing, a Percentage Calculator can be used to calculate the percentage of a discount on a product or service, or to determine the percentage increase in sales revenue over a period of time.
- In Science and Engineering: In science and engineering, a Percentage Calculator can be used to calculate the percentage error in measurements, or to determine the percentage change in a physical property such as temperature, pressure, or volume.
- In Health and Fitness: In health and fitness, a Percentage Calculator can be used to calculate the percentage of body fat or muscle mass, or to determine the percentage change in weight or BMI (body mass index) over a period of time.
- In Cooking and Nutrition: In cooking and nutrition, a Percentage Calculator can be used to calculate the percentage of a nutrient or ingredient in a recipe, or to determine the percentage increase or decrease in calorie intake.
Overall, the Percentage Calculator is a versatile tool that can be used in many different fields and applications to simplify and speed up calculations that involve percentages. The JavaScript Percentage Calculator project is a great way to learn how to build a tool that can be used in various real-world scenarios.
Design JavaScript Simple Projects Percentage Calculator in HTML CSS
Here are the steps to design a Percentage Calculator in HTML and CSS:
Step 1: Create a new HTML documentĀ
Create a new HTML document in a text editor or integrated development environment (IDE) such as Visual Studio Code or Atom.
Step 2: Set up the HTML documentĀ
Add the basic structure of an HTML document, including the doctype, html, head, and body elements. Inside the head element, add a title for the page.
Step 3: Create a form elementĀ
Inside the body element, create a form element with a unique ID. This form will contain the input fields and buttons for the Percentage Calculator.
Step 4: Add input fields for numbersĀ
Within the form element, create two input fields for numbers that will be used to calculate the percentage. Assign unique IDs to each input field so they can be accessed by JavaScript. Add labels for each input field to provide a user-friendly interface.
Step 5: Create a select dropdown menuĀ
Create a select element with a unique ID that will be used to select the percentage calculation method (percentage increase or percentage decrease). Add two options to the selected element with values that correspond to the calculation method.
Step 6: Create a button to calculate the percentageĀ
Create a button element with a unique ID and add a label to it, such as "Calculate Percentage".
Step 7: Add styling with CSSĀ
Add CSS styling to the HTML elements to improve the appearance of the Percentage Calculator. You can use CSS properties such as background color, font size, padding, and border.
Step 8: Write JavaScript codeĀ
Write JavaScript code to calculate the percentage based on the user input and the selected calculation method. You can use built-in JavaScript methods such as parseFloat() and toFixed() to perform the calculation and format the result.
Step 9: Link the JavaScript file to the HTML documentĀ
Link the JavaScript file to the HTML document using the script tag. Add the link to the bottom of the HTML document, just before the closing body tag.
Step 10: Test and refineĀ
Test the Percentage Calculator in a web browser and make any necessary refinements to the design or functionality.
That's it! With these steps, you can create a simple Percentage Calculator in HTML and CSS. You can always add more advanced features and styling to the Calculator to make it more user-friendly and visually appealing.
Ā
< html>
< head>
< title>Percentage Calculator< /title>
< /head>
< body>
< div class="container">
< h1>Percentage Calculator< /h1>
< div class="input-field">
< label for="num1">Number 1:< /label>
< input type="number" id="num1">
< /div>
< div class="input-field">
< label for="num2">Number 2:< /label>
< input type="number" id="num2">
< /div>
< button onclick="calculate()">Calculate Percentage< /button>
< div id="result">< /div>
< /div>
< script src="script.js">< /script>
< /body>
< /html>
.container {
width: 50%;
margin: 0 auto;
text-align: center;
}
.input-field {
margin: 20px 0;
}
label {
display: inline-block;
width: 100px;
text-align: left;
}
input[type="number"] {
width: 200px;
padding: 5px;
}
button {
padding: 5px 10px;
background-color: #4CAF50;
color: white;
border: none;
border-radius: 5px;
cursor: pointer;
}
#result {
margin: 20px 0;
}
This HTML code creates a basic structure of the percentage calculator with two input fields for entering numbers and a button to calculate the percentage. The calculation result will be displayed in the "result" div element. The button calls a function named "calculate" that should be defined in an external JavaScript file. The code creates a simple web page with two input fields to take the two numbers, and a button to calculate the percentage. The div with id="result" will show the result of the calculation.Ā
Ā
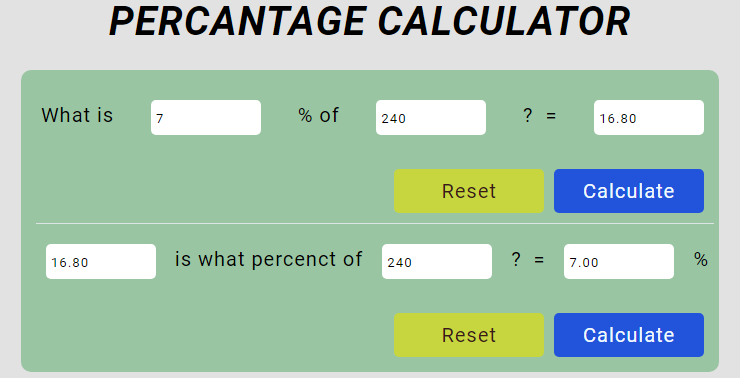
Ā
JavaScript Simple Projects Percentage Calculator Example Code
Here is the JavaScript code for the percentage calculator with an explanation:
function calculate() {
var num1 = document.getElementById("num1").value;
var num2 = document.getElementById("num2").value;
var result = document.getElementById("result");
if (num1 > 0) {
var percentage = (num2 * num1) / 100;
result.innerHTML = "The percentage is: " + percentage.toFixed(2) + "%";
} else {
result.innerHTML = "Number 1 should be greater than 0";
}
}
This is a JavaScript function named "calculate" that is used to calculate the percentage value based on the input values of two numbers. Here is how this function works:
- The function starts by getting the values of the two input fields with the IDs "num1" and "num2". These input fields are assumed to be numeric values entered by the user.
- Next, the function checks if the value of "num1" is greater than 0 using a simple if statement. This check is necessary to ensure that the percentage value is calculated correctly.
- If "num1" is greater than 0, the function calculates the percentage value using the formula (num2 * num1) / 100, where "num2" is the number for which the percentage value is to be calculated, and "num1" is the percentage value itself.
- The "toFixed()" method is used to round the percentage value to two decimal places and the resulting value is concatenated with a string "The percentage is: ".
- If "num1" is not greater than 0, the function sets the value of the "result" element to a string "Number 1 should be greater than 0".
- Finally, the value of "result" element is set to the calculated percentage value or the error message, depending on the input values.
Overall, this JavaScript function provides a simple and straightforward way to calculate the percentage value based on the input values of two numbers. It is suitable for beginners who are just starting to learn JavaScript and want to create a Percentage Calculator.
How this project will help beginners to learn javascript
This project will help beginners to learn JavaScript by providing a practical example of how JavaScript can be used to create a simple tool like a percentage calculator. It covers important concepts such as variables, functions, conditions, and DOM manipulation. Beginners can also explore additional features that can be added to the project to enhance their understanding of JavaScript.
Here are three percentage calculation examples that can be done using this calculator:
Example 1: Find the percentage increase of a number from 100 to 12.Ā
Number 1: 100Ā
Number 2: 20%Ā
Result: The percentage is: 20.00
Example 2: Find the percentage decrease of a number from 120 to 10Ā
Number 1: 120Ā
Number 2: 10%Ā
Result: The percentage is: 12.00
Conclusion
In this post, we learned how to create a percentage calculator using JavaScript. We discussed the design and code for the calculator and explored how to validate corner cases. We also provided examples of percentage calculations and additional features that can be added to the project. This project can be a great learning experience for beginners to understand the basics of JavaScript and create a practical tool.
Still you face problems, feel free to contact with me, I will try my best to help you.