JavaScript Registration Form Validation With free Source Code
Last Updated: 2023-06-18 01:30:40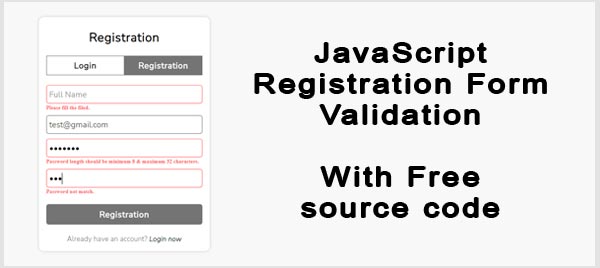
Registration form validation is an important process in web development, as it helps to ensure that the user's input is complete and accurate. This can be especially important when collecting sensitive information, such as personal or financial details.
In JavaScript, there are various techniques that can be used to validate a form, including using regular expressions, conditional statements, and built-in form validation functions.
In this tutorial, we will demonstrate how to create a simple registration form and validate it using JavaScript. The source code for this tutorial will be provided so that you can follow along and learn by example.
What is Registration Form Validation
Registration form validation is the process of checking that a user's input in a registration form is complete, accurate, and valid before the form is submitted. This can be especially important when collecting sensitive information, such as personal or financial details.
Validation can be used to ensure that all required fields are filled out, that input is in the correct format (such as an email address or phone number), and that input meets certain criteria (such as a password meeting minimum length requirements).
By performing validation checks, we can improve the user experience by providing helpful error messages and preventing invalid or incomplete data from being submitted to the server. This can help to prevent issues with data integrity and security. Please watch the video for a better understanding. Also, you can learn how to design and validate a login form using javascript.
Steps of Registration Form Validation in Javascript
Here are the general steps that you can follow to perform registration form validation in JavaScript:
- Create the HTML form: First, create the HTML registration form that will collect the user's input. Be sure to include all required form controls and give each control a unique name attribute.
- Add JavaScript to validate the form: Next, add JavaScript to the page that will validate the form when it is submitted. This can be done by attaching an event listener to the form's submit event and writing a function to perform the validation.
- Check required fields: One of the first things to check is that all required fields are filled out. You can use the HTML5 required attribute to mark required fields, or you can check for empty fields using JavaScript.
- Validate the input format: Check that the input is in the correct format. For example, you can use regular expressions to check that an email address is properly formatted or that a phone number contains only digits.
- Check that input meets criteria: Validate that the input meets any additional criteria that you have set. For example, you may require a password to be at least 8 characters long or to contain at least one uppercase letter.
- Display error messages: If any errors are found during validation, display appropriate error messages to the user.
- Submit the form if validation is successful: If the form passes validation, submit the form to the server for processing.
Note: These steps are just a general outline and the specific details of your validation code will depend on the specific requirements of your form.
Registration Form Validation in JavaScript Example Code
Suppose you have a simple registration form that has the Name, Email, Password, and Confirm Password fields. Now if you want to validate those filed using javascript you can write a javascript function like this.
Note: You can download the source code of a complete registration form validation in javascript from here.
function validateForm() {
// Get the form values
var name = document.forms["registrationForm"]["name"].value;
var email = document.forms["registrationForm"]["email"].value;
var password = document.forms["registrationForm"]["password"].value;
var confirmPassword = document.forms["registrationForm"]["confirmPassword"].value;
// Check that all required fields are filled out
if (name == "" || email == "" || password == "" || confirmPassword == "") {
alert("All fields are required. Please complete the form.");
return false;
}
// Check that the email is properly formatted
if (!/^\w+([\.-]?\w+)*@\w+([\.-]?\w+)*(\.\w{2,3})+$/.test(email)) {
alert("Please enter a valid email address.");
return false;
}
// Check that the password and confirm password fields match
if (password != confirmPassword) {
alert("The password and confirm password fields do not match.");
return false;
}
// If all validation checks pass, submit the form
return true;
}
This function does the following:
- It gets the values of the form fields using the document. forms object and the name attributes of the form controls.
- It checks that all required fields are filled out using an if statement. If any fields are empty, it displays an alert and returns false to prevent the form from being submitted.
- It uses a regular expression to check that the email is properly formatted. If the email is invalid, it displays an alert and returns false.
- It will checks that the password and confirm password fields match. If they do not match, it displays an alert and returns false.
- If all validation checks pass, it returns true, which allows the form to be submitted.
To use this function, you would need to attach it to the form's submit event like this:
< form name="registrationForm" onsubmit="return validateForm()">
< !-- form fields go here -->
< /form>
This will cause the validateForm function to be called whenever the form is submitted. If the function returns true, the form will be submitted. If it returns false, the form will not be submitted and the user will be shown any error messages that are displayed by the function. Just like the same way you can also validate your login form using javascript
Best practice for Registration Form Validation
There are a few best practices that you should follow when performing registration form validation:
Use client-side validation: Client-side validation is a validation that is performed in the user's web browser, rather than on the server. This can help to reduce the load on the server and improve the user experience by providing instant feedback on invalid input.
Use server-side validation: While client-side validation is important, it is also important to perform server-side validation to ensure that the data is valid and secure. This is because an attacker can bypass client-side validation, so it is important to double-check the data on the server.
Provide clear and concise error messages: If a user's input is invalid, it is important to provide clear and concise error messages that explain the issue and how to fix it. This will help the user to understand what went wrong and how to correct their input.
Use appropriate form controls: Use the proper form controls for the type of input you are collecting. For example, use a text box for short free-form text, a dropdown list for a list of options, and a checkbox for a binary yes/no choice.
Use built-in form validation functions: Many programming languages, including JavaScript, have built-in functions for performing common form validation tasks. These can be useful for quickly and easily validating input without having to write your own validation code.
The Benefits of Registration Form Validation in JavaScript
There are several reasons why you might want to validate a registration form using JavaScript:
Improved user experience: Validation can help to improve the user experience by providing instant feedback on invalid input. This can help the user to understand what went wrong and how to correct their input, rather than having to wait for the form to be submitted and receiving an error message from the server.
Data integrity: Validation can help to ensure that the data being submitted is complete, accurate, and in the correct format. This can help to prevent issues with data integrity and ensure that the data being stored in the database is reliable.
Security: Validation can help to prevent malicious input from being submitted to the server. For example, you can use validation to ensure that passwords meet minimum length requirements or that email addresses are properly formatted.
Reduced load on the server: By performing validation on the client side (in the user's web browser), you can reduce the load on the server by only sending valid data to the server for processing. This can help to improve the overall performance of your application.
Overall, validation is an important aspect of web development and can help to ensure that your application is reliable, secure, and easy to use.
The common mistake of Registration Form using javascript
Here are a few common mistakes that developers might make when creating a registration form with JavaScript:
Not performing server-side validation: It is important to perform both client-side and server-side validation to ensure that the data is secure and accurate. Client-side validation can be bypassed by an attacker, so it is important to double-check the data on the server.
Not providing clear error messages: If a user's input is invalid, it is important to provide clear and concise error messages that explain the issue and how to fix it. Without clear error messages, the user may not understand what went wrong and how to correct their input.
Not using appropriate form controls: Use the proper form controls for the type of input you are collecting. For example, use a text box for short free-form text, a dropdown list for a list of options, and a checkbox for a binary yes/no choice.
Not validating password strength: It is important to ensure that users choose strong passwords to protect their accounts. You can use JavaScript to check that passwords meet certain criteria, such as minimum length and the inclusion of uppercase letters, lowercase letters, and numbers.
Not handling special characters properly: If your form allows users to enter special characters, be sure to handle them properly. Some special characters, such as < and >, have special meanings in HTML and can cause issues if they are not properly encoded.
Overall, it is important to carefully plan and test your form validation code to ensure that it is effective and user-friendly.
Security Concern of Registration Form using javascript
There are a few security concerns that you should be aware of when creating a registration form with JavaScript:
Client-side validation can be bypassed: While client-side validation can be useful for improving the user experience and reducing the load on the server, it is important to remember that it can be bypassed by an attacker. It is important to also perform server-side validation to ensure that the data is secure.
Sensitive data should be encrypted: If your form collects sensitive data, such as passwords or financial information, it is important to ensure that this data is encrypted when it is transmitted to the server. This can help to protect against man-in-the-middle attacks and other forms of data interception.
Use appropriate form controls: Use the appropriate form controls for the type of input you are collecting. For example, use a password field for passwords and a hidden field for sensitive data that should not be displayed to the user.
Validate password strength: It is important to ensure that users choose strong passwords to protect their accounts. You can use JavaScript to check that passwords meet certain criteria, such as minimum length and the inclusion of uppercase letters, lowercase letters, and numbers.
Handle special characters properly: If your form allows users to enter special characters, be sure to handle them properly. Some special characters, such as < and >, have special meanings in HTML and can cause issues if they are not properly encoded.
By following these best practices, you can help to ensure the security of your registration form and protect the sensitive data that it collects.
Conclusion
In summary, registration form validation is an important process in web development that helps to ensure that the user's input is complete, accurate, and valid before the form is submitted. JavaScript is a powerful language that provides a variety of techniques for performing form validation, including using regular expressions, conditional statements, and built-in form validation functions.
By following best practices and taking security concerns into account, you can create a user-friendly and secure registration form that will help to improve the overall user experience of your application.
Still you face problems, feel free to contact with me, I will try my best to help you.